.mouseenter( handler )返回类型:jQuery
描述:绑定一个要在鼠标进入元素时引发的事件处理函数,或者在元素上触发此处理函数。
-
增补版本:1.0.mouseenter( handler )
-
handler每次触发事件时要执行的函数。
-
-
增补版本:1.4.3.mouseenter( [eventData ], handler )
-
eventData类型:Anything一个对象,包含要传递给事件处理函数的数据。
-
handler每次触发事件时要执行的函数。
-
-
增补版本:1.0.mouseenter()
- 此签名没有任何参数。
此方法在前两种变体中是.on( "mouseenter", handler )
的简写,在第三种变体中是.trigger( "mouseenter" )
的简写。
mouseenter
JavaScript事件是Internet Explorer 专有的。因为此事件通常很有用,jQuery模拟了此事件,从而它可用于所有浏览器。当鼠标指针进入一个元素时,此事件发送到该元素。任何HTML元素都可以接收此事件。
例如:考虑以下HTML:
1
2
3
4
5
6
7
8
9
10
|
|
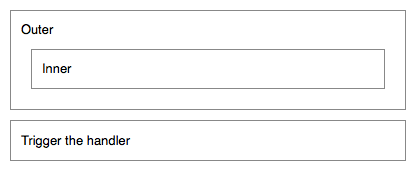
该事件处理函数可以绑定到任何元素:
1
2
3
|
|
现在当鼠标指针移到 Outer<div>上面时,消息追加到<div id="log">
后面。也可以在点击别的元素时触发此事件。
1
2
3
|
|
执行此代码之后,点击Trigger the handler也将追加消息。
mouseenter
事件处理事件冒泡的方式不同于mouseover
。如果此示例中使用了mouseover
,则当鼠标指针移到Inner元素上面时,将触发处理函数。这通常是不想要的行为。另一方面,mouseenter
只在鼠标进入它绑定的元素时才触发它的处理函数,进入后代元素时不触发。所以在此示例中,当鼠标进入Outer元素时,触发事件处理函数,但是进入Inner元素时不触发。
补充说明:
-
因为
.mouseenter()
方法是.on( "mouseenter", handler )
的简写,所以可以使用.off( "mouseenter" )
来分离。
示例:
当触发mouseenter事件和mouseout事件时显示文本。
当指针移到子元素里面时,也引发mouseover
事件,与此同时,只有当鼠标指针移入绑定元素时,才引发mouseenter
事件。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
|
|